
The nullish coalescing operator (??
) is a logical operator that returns its right-hand side operand when its left-hand side operand is null
or undefined
, and otherwise returns its left-hand side operand.
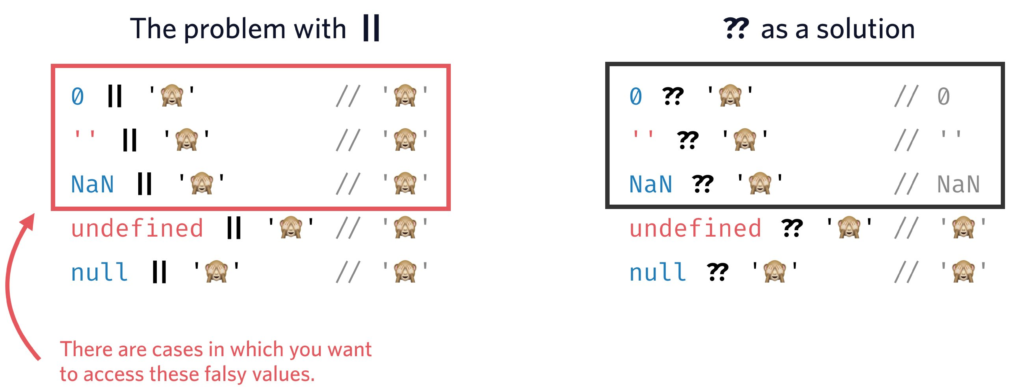
?. → Optional Chaining operator
Optional chaining will eliminate the need for manually checking if a property is available in an object. With option chaining the checking will be done internally.
Example without Optional chaining.
const planet1 = {
name: 'Earth',
size: '6,371 km'
}
const planet2 = {
name: 'Mars',
// size: '3,389.5 km'
}
function printPlanetInfo(planet) {
console.log(`Name: ${planet.name}`)
console.log(`Size: ${planet.size.toUpperCase()}`)
}
printPlanetInfo(planet1)
printPlanetInfo(planet2)
When we pass an planet object which doesn’t have the size property:
To solve the above problem what we do is, we will add check if size property available in the planet object
const planet1 = {
name: 'Earth',
size: '6,371 km'
}
const planet2 = {
name: 'Mars',
// size: '3,389.5 km'
}
function printPlanetInfo(planet) {
console.log(`Name: ${planet.name}`)
planet.size = ( planet && planet.size &&
planet.size.toUpperCase())
|| "undefined"
console.log(`Size: ${planet.size}`)
}
printPlanetInfo(planet1)
printPlanetInfo(planet2)
The optional chaining will check if an object left to the operator is valid (not null and undefined). If the property is valid then it executes the right side part of the operator otherwise return undefined.
function printPlanetInfo(planet) {
console.log(`Name: ${planet.name}`)
planet.size = ( planet?.size?.toUpperCase() ) || "undefined"
console.log(`Size: ${planet.size}`)
}
The native JavaScript equivalent code for above optional chaining operator is
(property == undefined || property == null) ? undefined : property
We can use variables as property name in optional chaining
const planet = {
name: 'Earth',
size: '6,371 km'
}
planet?.size
// We can also use with expressions
planet?.["s"+"ize"]
You can use optional chaining to call a method which may not exist.
const planet = {
name: 'Earth',
size: '6,371 km'
getSize() {
return this.size;
}
}
planet?.getSize?.()
Reference: MDN.
Make it run without errors but you cannot change the location of the `let` statement, that has to stay at the end.
This was a trick question.
Callbacks are not async by default, this was a sync call so the message var was not defined by the time the callback was called.To make this work we need to wrap our call to `cb` in either a `setImmediate` or a `process.nextTick`.